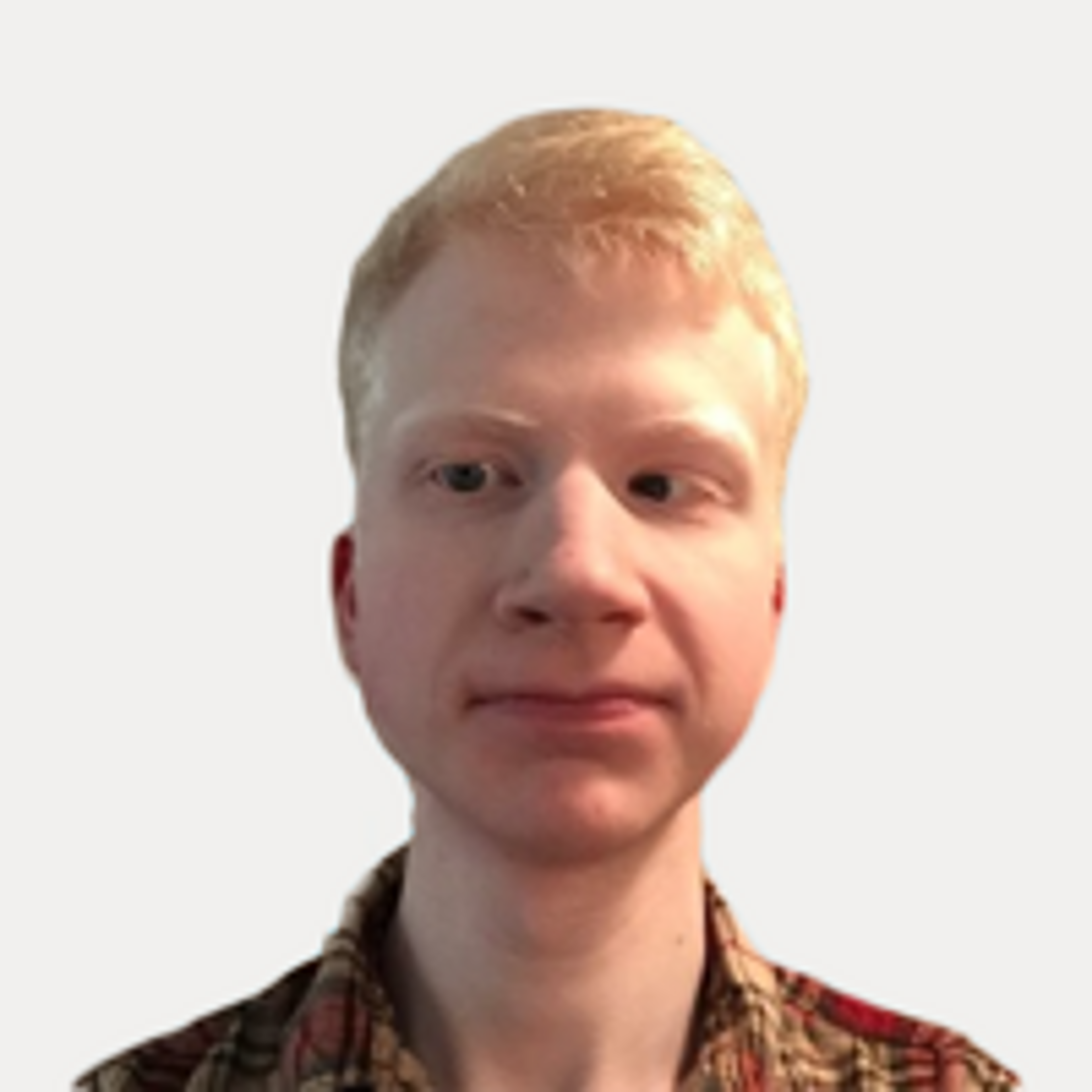
Owen LaRosa
Instructor
Owen is an iOS and Android app developer, and is the Student Experience Lead for iOS programs at Udacity. He graduated from the iOS Developer Nanodegree program in 2015.
Nanodegree Program
Master writing in Swift as you build five portfolio-worthy iOS apps to demonstrate your expertise as an iOS Developer
Master writing in Swift as you build five portfolio-worthy iOS apps to demonstrate your expertise as an iOS Developer
Intermediate
5 months
Real-world Projects
Completion Certificate
Last Updated August 9, 2023
Course 1 • 32 minutes
Course 2 • 18 hours
Learn the basics of Swift, the programming language used to develop iOS apps.
Course 3 • 4 weeks
Build your first app with Swift and Xcode, Apple’s programming environment for app development.
Course 4 • 1 month
Develop an app with UIKit, Apple’s front-end framework for developing fast and powerful web interfaces.
Instructor
Owen is an iOS and Android app developer, and is the Student Experience Lead for iOS programs at Udacity. He graduated from the iOS Developer Nanodegree program in 2015.
Instructor
Kate is an iOS developer, speaker, author, and teacher who has spoken at conferences across the globe from AltConf in San Francisco to Mobile Central Europe in Poland. She also has hosted a podcast on work-life integration for parents in tech.
Instructor
Gabrielle earned her Ph.D. in Population Biology from UC Davis, where she discovered the joys of programming while analyzing DNA sequences. She has a background in teaching, and worked as an iOS Engineer before joining Udacity.
Instructor
Jarrod is an experienced iOS developer with a passion for reinventing how students learn. He holds a BS in Computer Science from the University of Alabama.
Average Rating: 4.6 Stars
225 Reviews
Combine technology training for employees with industry experts, mentors, and projects, for critical thinking that pushes innovation. Our proven upskilling system goes after success—relentlessly.
Demonstrate proficiency with practical projects
Projects are based on real-world scenarios and challenges, allowing you to apply the skills you learn to practical situations, while giving you real hands-on experience.
Gain proven experience
Retain knowledge longer
Apply new skills immediately
Top-tier services to ensure learner success
Reviewers provide timely and constructive feedback on your project submissions, highlighting areas of improvement and offering practical tips to enhance your work.
Get help from subject matter experts
Learn industry best practices
Gain valuable insights and improve your skills
Unlimited access to our top-rated courses
Real-world projects
Personalized project reviews
Program certificates
Proven career outcomes
Full Catalog Access
One subscription opens up this course and our entire catalog of projects and skills.
Average time to complete a Nanodegree program
iOS Developer