
Strings are a building block of most programming languages, and Python is no exception. In fact, one of the first skills you’ll need when picking up Python is how to work with strings. There are many ways to have your program evaluate and print strings with injected values. However, not every project is as simple as printing out a fixed “Hello, World.” In this tutorial, we’ll learn three methods of Python string formatting that use variables, inbuilt methods and special symbols.
What Is String Formatting?
Unlike printing out a fixed string like “Hello, World,” string formatting requires interpolating values within a provided string, as in “Hello <variable>.” In the latter, the variable is substituted at runtime with a person’s name. This means you can inject variables within a string that, before interpolation, contains the placeholders in which the values will eventually live.
There are a few valid Python string formatting methods. We have the common str.format() along with the even newer f-string, which debuted with Python 3.6. A less utilized but still standard way of formatting strings involves the modulo operator (the percent sign) followed by a conversion type to indicate a special type of format.
In this article, we’ll focus on Python string formatting using newer methods with brief explanations of the Python 2 modulo approach.
Using str.format()
The Python format function has been the standard way to format strings since the later versions of Python 2 were released. It used to be the “new” way to format strings until the f-string appeared with Python 3.6. Using this method, you can achieve your intended result by using curly braces and supplying values to the .format() function:

The variable that you use to call format() is known as a formatter. You can use a single formatter as shown above, or multiple formatters, as follows:

The formatters don’t even need to be strings — we can use integers, floats and other data types. One nice benefit of using str.format() is that, unlike with modulos, we don’t need to specify the type of the variables we provide as formatters. Python figures out what data type the variables are and injects them appropriately:

What we’ve seen so far involves positional arguments: We provide the to-be-inserted variables in the order we want them positioned in the string. This is because tuples (the data type containing the variables) are indexed, meaning they have a preset order. Python thus figures out which variable corresponds to which pair of curly braces, but you can explicitly provide positional arguments if you wish:

Although you don’t need to supply positional arguments when your variables are in order, the tuple index is always associated with its value, which lets you access them dynamically within your given string. For example:

In case keeping track of what goes where gets confusing, you can always use keyword arguments, as below:

As you can see, you’re able to use a mix of positional and keyword arguments. And though we established that you don’t need type specifiers, these can still be handy in some cases, including for rounding numbers. Here we use “.0f” to denote a float number without any decimal points:

You can even pass objects to the values in your tuple, as below:

Or more succinctly:

It’s clear that str.format() gives you plenty of dynamic options when using strings! However, using the format function can still be pretty verbose even while providing more readability than the modulo operator (which we’ll look at in a bit). Let’s look at f-strings and see if they improve our readability.
The f-string (Formatted String Literal)
Back in 2015, Python introduced the formatted string literal, commonly called the f-string. You can easily recognize an f-string, as it always begins with a lower-case or capital f! F-strings are special in that you can embed Python expressions in curly braces directly within your string. Here’s our example:

Already, you can see that f-strings are quite readable. If you have a long string with many variables, you can space them over multiple lines to maintain readability:
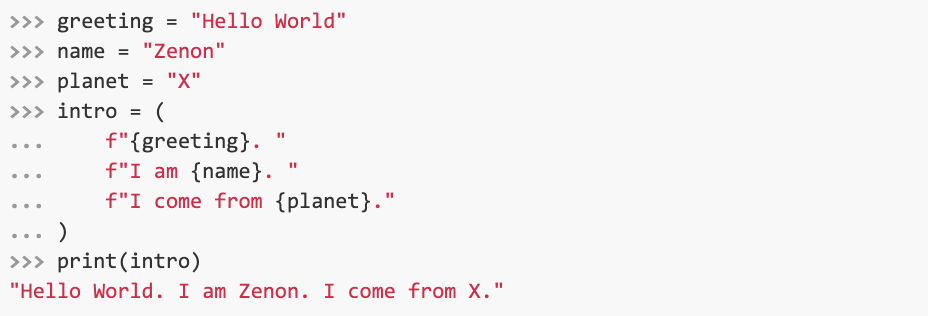
You can do fancy things with f-strings, including calling inbuilt methods, custom functions and objects from your own classes. Here, we use inbuilt function min():

If you want fast string formatting, f-strings are for you. Their speed advantage owes to the fact that they’re evaluated at runtime, seeing as they are expressions and not fixed values.
There are a couple of key limitations to using f-strings. First, you need to have Python 3.6 installed, which means you can’t use them when working on projects with older source code. You also need to be careful with syntax; f-strings can be picky with quotations, for example, and mistakes are common. With that said, let’s now look at the original — and now outdated — method of Python string formatting.
Modulo Operator
The Python modulo operator covers the same functionality as string.Template. It’s important to know because you’ll likely see it in older code you might be working with. Also, if you know languages like C, modulo operators might be an ideal entry-point for you in terms of learning Python string formatting, as they’re nearly identical to C’s printf format string functions.
Here’s a simple example of how to use the modulo operator:

The conversion specifier %s in the format string acts as a placeholder for the value(s) that follow the % symbol, much in the same way that curly braces placehold in the str.format(). Here, the specifier ‘s’ denotes that the value ‘World’ is a string. Of course, you can use other type specifiers to reference other data types. These specifiers tell Python how to insert the specified values.
As we mentioned, the modulo operator works in the same way as the Template class of the String module, just with different syntax. At the time that developers were heavily relying on the modulo operator, template strings became popular for international purposes, since the syntax made it easier to translate to speakers of other languages. In Python string formatting, both approaches are now less favored in comparison to the format function and f-strings.
If you’d like to learn more about the modulo operator, we cover it in depth in this blog post.
Learn More
String formatting is one of many essential skills required of any Python developer. Master not only Python string formatting but other core programming concepts with our expert-taught Introduction to Programming Nanodegree.