Are you looking to learn more about the getline function in C++? We’ve got you covered. In this article, we walk through a few usage examples for getline and explain how it differs from the commonly used operator.
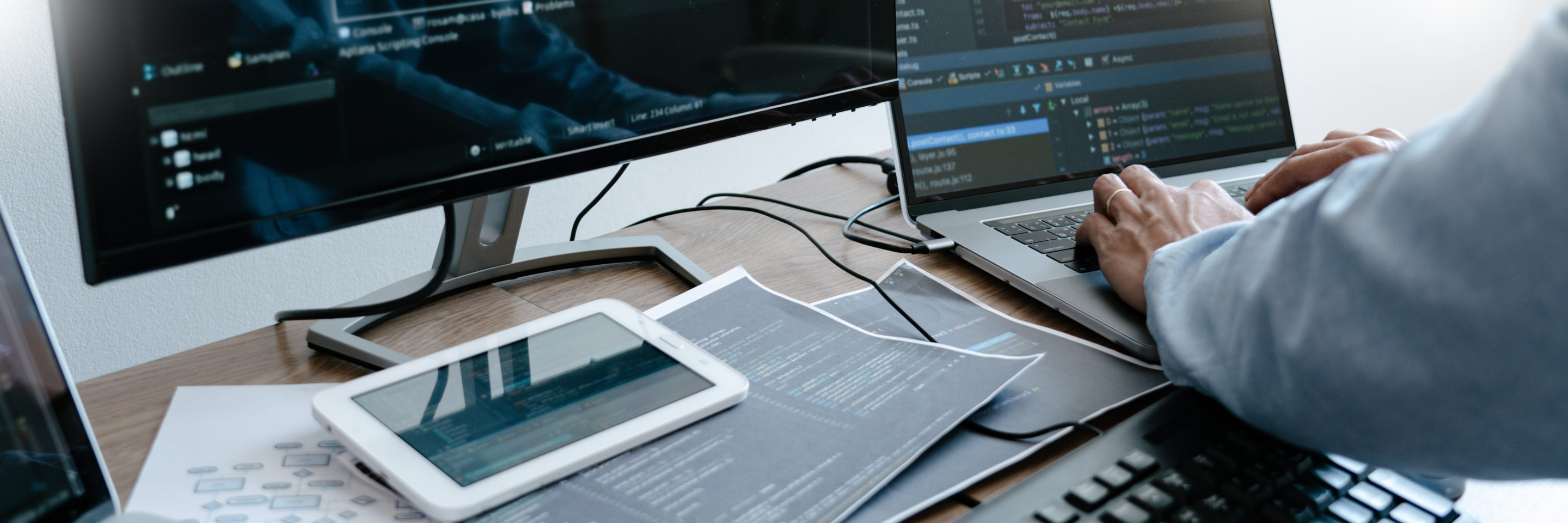
What is getline in C++?
In C++, the getline function is a way to read an entire line of console input into a variable. Here is a simple example of reading input using getline:
#include <iostream>
#include <string>
using namespace std;
int main() {
string name;
cout << "Please enter your name" << endl;
getline(cin, name);
cout << "Hi, " << name << "!" << endl;
}
The getline function takes an input stream and a string as parameters (cin is console input in the example above) and reads a line of text from the stream into the string.
getline is easier to use for reading sequences of inputs than other functions such as the >> operator, which is more oriented toward words or characters. Because of this flexibility, developers frequently rely on getline for processing user input.
C++ getline use cases
The primary use case for getline is reading from an input stream in your C++ program. Frequently, the stream you need to read from is standard input (stdin), where a user types in the information line by line, separated by newline characters (\n). Your application then stores the user input using a data structure like a C++ map or an array.
You can find this use case in any program that receives external information in text form, from text parsers and compilers to accounting software and word processors.
We see a variation on this primary use case for getline when reading input to your program from a text file. Linux, macOS and other UNIX-like operating systems support redirecting the contents of a file into a program’s standard input. Let’s say your input is in a text file, helpfully named input.txt, and your executable is called program.x. You can easily redirect the text file’s contents to program.x ’s input by using the < symbol in the system shell:
$ ./program.x < input.txt
When passing input to the program this way, you can also use getline—with no changes to the application required—to read the file’s contents. Keep in mind, however, that this approach to reading files is not very robust and has many limitations, such as only being able to read a single file at a time and requiring the user to pass the data in when executing the program. If you really want to read input from files in your C++ program, consider using C++ file primitives instead of using getline.
How do I use getline in C++?
If you’re aiming to read user input from the console using getline, the code for this task might look something like this:
#include <iostream>
#include <string>
using namespace std;
int main() {
string name;
cout << "Please enter your name" << endl;
getline(cin, name);
cout << "Hi, " << name << "!" << endl;
}
In this example, we start by declaring the string variable that will hold the input read in by getline. We then use getline to read the text into the variable and print out a greeting message.
Now, you might also want to use getline to read all the input available through the console rather than a single line. Look at this example of reading lines in a loop:
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
int main() {
string line;
map
getline(cin, line);
while (!line.empty()) {
// convert the line to upper case
transform(line.begin(), line.end(), line.begin(), ::toupper);
getline(cin, line);
}
}
In each loop iteration, the example above checks the length of the line it has received. Should the line only contain the end-of-line character, since any delimiting characters get removed, the length of the string getline reads is zero. That causes the loop to exit once the program has read all available input. This code assumes that the data does’t contain any blank lines.
What’s the difference between getline and the extraction operator?
You may well have used the extraction operator( >>) before to read values from standard input:
...
int a;
string b;
cin >> a >> b;
...
You can also use this operator to read user input. So if you’re wondering, “Why not just use >> instead of getline?”, here’s a caveat: when reading strings, the >> operator interprets all whitespace as the end of a string to read, which means that spaces, tabs, and newlines all act as input separators. Therefore it mainly makes sense to use the >> operator for reading words rather than entire lines of text.
getline, in contrast, reads until the line end by default and does not count spaces or tabs as line separators (although you can configure getline to use a different line-separator character, such as tab or space). This feature of getline means that you can read entire lines of input more quickly.
Further reading and resources
In this article, we walked you through the basics of using the C++ getline function. Check out the getline reference for more details on available options and links to related functions.
If you’d like to learn more about C++, enroll in our C++ Nanodegree program.